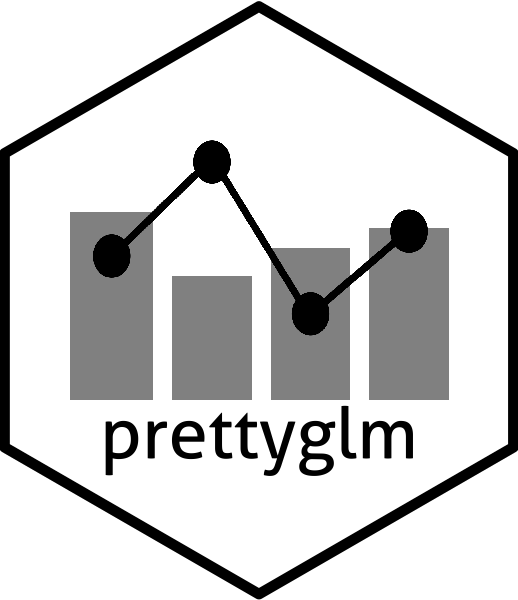
pretty_relativities
pretty_relativities.Rd
Creates a pretty html plot of model relativities including base Levels.
Usage
pretty_relativities(
feature_to_plot,
model_object,
plot_approx_ci = TRUE,
relativity_transform = "exp(estimate)-1",
relativity_label = "Relativity",
ordering = NULL,
plot_factor_as_numeric = FALSE,
width = 800,
height = 500,
iteractionplottype = NULL,
facetorcolourby = NULL,
upper_percentile_to_cut = 0.01,
lower_percentile_to_cut = 0,
spline_seperator = NULL
)
Arguments
- feature_to_plot
A string of the variable to plot.
- model_object
Model object to create coefficient table for. Must be of type: glm, lm
- plot_approx_ci
Set to TRUE to include confidence intervals in summary table. Warning, can be computationally expensive.
- relativity_transform
String of the function to be applied to the model estimate to calculate the relativity, for example: 'exp(estimate)'. Default is for relativity to be 'exp(estimate)-1'.
- relativity_label
String of label to give to relativity column if you want to change the title to your use case, some users may prefer to refer to this as odds ratio.
- ordering
Option to change the ordering of categories on the x axis, only for discrete categories. Default to the ordering of the fitted factor. Other options are: 'alphabetical', 'Number of records', 'Average Value'
- plot_factor_as_numeric
Set to TRUE to return data.frame instead of creating kable.
- width
Width of plot
- height
Height of plot
- iteractionplottype
If plotting the relativity for an interaction variable you can "facet" or "colour" by one of the interaction variables. Defaults to null.
- facetorcolourby
If iteractionplottype is not Null, then this is the variable in the interaction you want to colour or facet by.
- upper_percentile_to_cut
For continuous variables this is what percentile to exclude from the upper end of the distribution. Defaults to 0.01, so the maximum percentile of the variable in the plot will be 0.99. Cutting off some of the distribution can help the views if outlier's are present in the data.
- lower_percentile_to_cut
For continuous variables this is what percentile to exclude from the lower end of the distribution. Defaults to 0.01, so the mimimum percentile of the variable in the plot will be 0.01. Cutting off some of the distribution can help the views if outlier's are present in the data.
- spline_seperator
string of the spline separator. For example AGE_0_25 would be "_".
Examples
library(dplyr)
library(prettyglm)
data('titanic')
columns_to_factor <- c('Pclass',
'Sex',
'Cabin',
'Embarked',
'Cabintype',
'Survived')
meanage <- base::mean(titanic$Age, na.rm=TRUE)
titanic <- titanic %>%
dplyr::mutate_at(columns_to_factor, list(~factor(.))) %>%
dplyr::mutate(Age =base::ifelse(is.na(Age)==TRUE,meanage,Age)) %>%
dplyr::mutate(Age_0_25 = prettyglm::splineit(Age,0,25),
Age_25_50 = prettyglm::splineit(Age,25,50),
Age_50_120 = prettyglm::splineit(Age,50,120)) %>%
dplyr::mutate(Fare_0_250 = prettyglm::splineit(Fare,0,250),
Fare_250_600 = prettyglm::splineit(Fare,250,600))
survival_model3 <- stats::glm(Survived ~
Pclass:Embarked +
Age_0_25 +
Age_25_50 +
Age_50_120 +
Sex:Fare_0_250 +
Sex:Fare_250_600 +
SibSp +
Parch +
Cabintype,
data = titanic,
family = binomial(link = 'logit'))
# categorical factor
pretty_relativities(feature_to_plot = 'Cabintype',
model_object = survival_model3)
# continuous factor
pretty_relativities(feature_to_plot = 'Parch',
model_object = survival_model3)
# splined continuous factor
pretty_relativities(feature_to_plot = 'Age',
model_object = survival_model3,
spline_seperator = '_',
upper_percentile_to_cut = 0.01,
lower_percentile_to_cut = 0.01)
# factor factor interaction
pretty_relativities(feature_to_plot = 'Pclass:Embarked',
model_object = survival_model3,
iteractionplottype = 'colour',
facetorcolourby = 'Pclass')
# Continuous spline and categorical by colour
pretty_relativities(feature_to_plot = 'Sex:Fare',
model_object = survival_model3,
spline_seperator = '_')
#> Factor and Continuous Spine interaction detected, defualting to iteractionplottype = colour
# Continuous spline and categorical by facet
pretty_relativities(feature_to_plot = 'Sex:Fare',
model_object = survival_model3,
spline_seperator = '_',
iteractionplottype = 'facet')