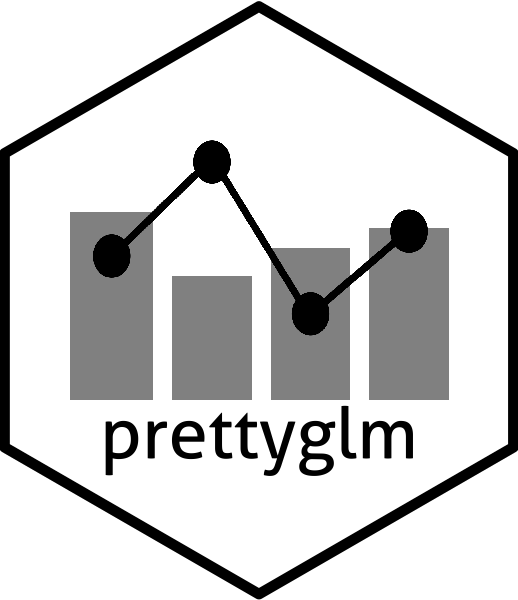
pretty_coefficients
pretty_coefficients.Rd
Creates a pretty kable of model coefficients including coefficient base levels, type III P.values, and variable importance.
Usage
pretty_coefficients(
model_object,
relativity_transform = NULL,
relativity_label = "relativity",
type_iii = NULL,
conf.int = FALSE,
vimethod = "model",
spline_seperator = NULL,
significance_level = 0.05,
return_data = FALSE,
...
)
Arguments
- model_object
Model object to create coefficient table for. Must be of type:
glm
,lm
.- relativity_transform
String of the function to be applied to the model estimate to calculate the relativity, for example: 'exp(estimate)-1'. Default is for relativity to be excluded from output.
- relativity_label
String of label to give to relativity column if you want to change the title to your use case.
- type_iii
Type III statistical test to perform. Default is none. Options are 'Wald' or 'LR'. Warning 'LR' can be computationally expensive. Test performed via
Anova
- conf.int
Set to TRUE to include confidence intervals in summary table. Warning, can be computationally expensive.
- vimethod
Variable importance method to pass to method of
vi
. Defaults to "model". Currently supports "permute" and "firm", pass any additional arguments tovi
in ...- spline_seperator
Separator to look for to identity a spline. If this input is not null, it is assumed any features with this separator are spline columns. For example an age spline from 0 to 25 you could use: AGE_0_25 and "_".
- significance_level
Significance level to P-values by in kable. Defaults to 0.05.
- return_data
Set to TRUE to return
data.frame
instead of creatingkable
.- ...
Any additional parameters to be past to
vi
Value
kable
if return_data = FALSE. data.frame
if return_data = TRUE.
Examples
library(dplyr)
library(prettyglm)
data('titanic')
columns_to_factor <- c('Pclass',
'Sex',
'Cabin',
'Embarked',
'Cabintype',
'Survived')
meanage <- base::mean(titanic$Age, na.rm=TRUE)
titanic <- titanic %>%
dplyr::mutate_at(columns_to_factor, list(~factor(.))) %>%
dplyr::mutate(Age =base::ifelse(is.na(Age)==TRUE,meanage,Age)) %>%
dplyr::mutate(Age_0_25 = prettyglm::splineit(Age,0,25),
Age_25_50 = prettyglm::splineit(Age,25,50),
Age_50_120 = prettyglm::splineit(Age,50,120)) %>%
dplyr::mutate(Fare_0_250 = prettyglm::splineit(Fare,0,250),
Fare_250_600 = prettyglm::splineit(Fare,250,600))
# A simple example
survival_model <- stats::glm(Survived ~
Pclass +
Sex +
Age +
Fare +
Embarked +
SibSp +
Parch +
Cabintype,
data = titanic,
family = binomial(link = 'logit'))
pretty_coefficients(survival_model)
#> <table class="table" style="margin-left: auto; margin-right: auto;border-bottom: 0;">
#> <thead>
#> <tr>
#> <th style="text-align:left;"> Variable </th>
#> <th style="text-align:left;"> Level </th>
#> <th style="text-align:left;"> Importance </th>
#> <th style="text-align:right;"> Estimate </th>
#> <th style="text-align:right;"> Std.error </th>
#> <th style="text-align:right;"> P.Value </th>
#> </tr>
#> </thead>
#> <tbody>
#> <tr>
#> <td style="text-align:left;"> (Intercept) </td>
#> <td style="text-align:left;"> (Intercept) </td>
#> <td style="text-align:left;"> <svg xmlns="http://www.w3.org/2000/svg" xmlns:xlink="http://www.w3.org/1999/xlink" class="svglite" width="48.00pt" height="12.00pt" viewbox="0 0 48.00 12.00"><defs><style type="text/css">
#> .svglite line, .svglite polyline, .svglite polygon, .svglite path, .svglite rect, .svglite circle {
#> fill: none;
#> stroke: #000000;
#> stroke-linecap: round;
#> stroke-linejoin: round;
#> stroke-miterlimit: 10.00;
#> }
#> .svglite text {
#> white-space: pre;
#> }
#> </style></defs><rect width="100%" height="100%" style="stroke: none; fill: none;"></rect><defs><clippath id="cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw"><rect x="0.00" y="0.00" width="48.00" height="12.00"></rect></clippath></defs><g clip-path="url(#cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw)"><polyline points="3.42,6.00 3.42,6.00 " style="stroke-width: 7.50; stroke: #D3D3D3;"></polyline><text x="24.00" y="66.72" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="38.87px" lengthadjust="spacingAndGlyphs">c(0, 0)</text><text transform="translate(-46.08,6.00) rotate(-90)" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="38.87px" lengthadjust="spacingAndGlyphs">c(1, 1)</text></g></svg>
#> </td>
#> <td style="text-align:right;"> 4.3534863 </td>
#> <td style="text-align:right;"> 0.7100919 </td>
#> <td style="text-align:right;"> <span style=" border-radius: 4px; padding-right: 4px; padding-left: 4px; background-color: #FFFFFF !important;">0</span> </td>
#> </tr>
#> <tr>
#> <td style="text-align:left;"> Pclass </td>
#> <td style="text-align:left;"> 1 </td>
#> <td style="text-align:left;"> <svg xmlns="http://www.w3.org/2000/svg" xmlns:xlink="http://www.w3.org/1999/xlink" class="svglite" width="48.00pt" height="12.00pt" viewbox="0 0 48.00 12.00"><defs><style type="text/css">
#> .svglite line, .svglite polyline, .svglite polygon, .svglite path, .svglite rect, .svglite circle {
#> fill: none;
#> stroke: #000000;
#> stroke-linecap: round;
#> stroke-linejoin: round;
#> stroke-miterlimit: 10.00;
#> }
#> .svglite text {
#> white-space: pre;
#> }
#> </style></defs><rect width="100%" height="100%" style="stroke: none; fill: none;"></rect><defs><clippath id="cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw"><rect x="0.00" y="0.00" width="48.00" height="12.00"></rect></clippath></defs><g clip-path="url(#cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw)"><polyline points="3.42,6.00 3.42,6.00 " style="stroke-width: 7.50; stroke: #D3D3D3;"></polyline><text x="24.00" y="66.72" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="38.87px" lengthadjust="spacingAndGlyphs">c(0, 0)</text><text transform="translate(-46.08,6.00) rotate(-90)" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="38.87px" lengthadjust="spacingAndGlyphs">c(1, 1)</text></g></svg>
#> </td>
#> <td style="text-align:right;"> 0.0000000 </td>
#> <td style="text-align:right;"> 0.0000000 </td>
#> <td style="text-align:right;"> <span style=" border-radius: 4px; padding-right: 4px; padding-left: 4px; background-color: #FFFFFF !important;">NA</span> </td>
#> </tr>
#> <tr>
#> <td style="text-align:left;"> Pclass </td>
#> <td style="text-align:left;"> 2 </td>
#> <td style="text-align:left;"> <svg xmlns="http://www.w3.org/2000/svg" xmlns:xlink="http://www.w3.org/1999/xlink" class="svglite" width="48.00pt" height="12.00pt" viewbox="0 0 48.00 12.00"><defs><style type="text/css">
#> .svglite line, .svglite polyline, .svglite polygon, .svglite path, .svglite rect, .svglite circle {
#> fill: none;
#> stroke: #000000;
#> stroke-linecap: round;
#> stroke-linejoin: round;
#> stroke-miterlimit: 10.00;
#> }
#> .svglite text {
#> white-space: pre;
#> }
#> </style></defs><rect width="100%" height="100%" style="stroke: none; fill: none;"></rect><defs><clippath id="cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw"><rect x="0.00" y="0.00" width="48.00" height="12.00"></rect></clippath></defs><g clip-path="url(#cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw)"><polyline points="3.42,6.00 5.13,6.00 " style="stroke-width: 7.50; stroke: #D3D3D3;"></polyline><text x="24.00" y="66.72" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="157.28px" lengthadjust="spacingAndGlyphs">c(0, 0.550292875865008)</text><text transform="translate(-46.08,6.00) rotate(-90)" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="38.87px" lengthadjust="spacingAndGlyphs">c(1, 1)</text></g></svg>
#> </td>
#> <td style="text-align:right;"> -0.2405205 </td>
#> <td style="text-align:right;"> 0.4370773 </td>
#> <td style="text-align:right;"> <span style=" border-radius: 4px; padding-right: 4px; padding-left: 4px; background-color: #F08080 !important;">0.58212</span> </td>
#> </tr>
#> <tr>
#> <td style="text-align:left;"> Pclass </td>
#> <td style="text-align:left;"> 3 </td>
#> <td style="text-align:left;"> <svg xmlns="http://www.w3.org/2000/svg" xmlns:xlink="http://www.w3.org/1999/xlink" class="svglite" width="48.00pt" height="12.00pt" viewbox="0 0 48.00 12.00"><defs><style type="text/css">
#> .svglite line, .svglite polyline, .svglite polygon, .svglite path, .svglite rect, .svglite circle {
#> fill: none;
#> stroke: #000000;
#> stroke-linecap: round;
#> stroke-linejoin: round;
#> stroke-miterlimit: 10.00;
#> }
#> .svglite text {
#> white-space: pre;
#> }
#> </style></defs><rect width="100%" height="100%" style="stroke: none; fill: none;"></rect><defs><clippath id="cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw"><rect x="0.00" y="0.00" width="48.00" height="12.00"></rect></clippath></defs><g clip-path="url(#cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw)"><polyline points="3.42,6.00 13.31,6.00 " style="stroke-width: 7.50; stroke: #D3D3D3;"></polyline><text x="24.00" y="66.72" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="149.64px" lengthadjust="spacingAndGlyphs">c(0, 3.19015516912915)</text><text transform="translate(-46.08,6.00) rotate(-90)" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="38.87px" lengthadjust="spacingAndGlyphs">c(1, 1)</text></g></svg>
#> </td>
#> <td style="text-align:right;"> -1.3950560 </td>
#> <td style="text-align:right;"> 0.4373004 </td>
#> <td style="text-align:right;"> <span style=" border-radius: 4px; padding-right: 4px; padding-left: 4px; background-color: #FFFFFF !important;">0.00142</span> </td>
#> </tr>
#> <tr>
#> <td style="text-align:left;"> Sex </td>
#> <td style="text-align:left;"> female </td>
#> <td style="text-align:left;"> <svg xmlns="http://www.w3.org/2000/svg" xmlns:xlink="http://www.w3.org/1999/xlink" class="svglite" width="48.00pt" height="12.00pt" viewbox="0 0 48.00 12.00"><defs><style type="text/css">
#> .svglite line, .svglite polyline, .svglite polygon, .svglite path, .svglite rect, .svglite circle {
#> fill: none;
#> stroke: #000000;
#> stroke-linecap: round;
#> stroke-linejoin: round;
#> stroke-miterlimit: 10.00;
#> }
#> .svglite text {
#> white-space: pre;
#> }
#> </style></defs><rect width="100%" height="100%" style="stroke: none; fill: none;"></rect><defs><clippath id="cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw"><rect x="0.00" y="0.00" width="48.00" height="12.00"></rect></clippath></defs><g clip-path="url(#cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw)"><polyline points="3.42,6.00 3.42,6.00 " style="stroke-width: 7.50; stroke: #D3D3D3;"></polyline><text x="24.00" y="66.72" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="38.87px" lengthadjust="spacingAndGlyphs">c(0, 0)</text><text transform="translate(-46.08,6.00) rotate(-90)" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="38.87px" lengthadjust="spacingAndGlyphs">c(1, 1)</text></g></svg>
#> </td>
#> <td style="text-align:right;"> 0.0000000 </td>
#> <td style="text-align:right;"> 0.0000000 </td>
#> <td style="text-align:right;"> <span style=" border-radius: 4px; padding-right: 4px; padding-left: 4px; background-color: #FFFFFF !important;">NA</span> </td>
#> </tr>
#> <tr>
#> <td style="text-align:left;"> Sex </td>
#> <td style="text-align:left;"> male </td>
#> <td style="text-align:left;"> <svg xmlns="http://www.w3.org/2000/svg" xmlns:xlink="http://www.w3.org/1999/xlink" class="svglite" width="48.00pt" height="12.00pt" viewbox="0 0 48.00 12.00"><defs><style type="text/css">
#> .svglite line, .svglite polyline, .svglite polygon, .svglite path, .svglite rect, .svglite circle {
#> fill: none;
#> stroke: #000000;
#> stroke-linecap: round;
#> stroke-linejoin: round;
#> stroke-miterlimit: 10.00;
#> }
#> .svglite text {
#> white-space: pre;
#> }
#> </style></defs><rect width="100%" height="100%" style="stroke: none; fill: none;"></rect><defs><clippath id="cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw"><rect x="0.00" y="0.00" width="48.00" height="12.00"></rect></clippath></defs><g clip-path="url(#cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw)"><polyline points="3.42,6.00 44.58,6.00 " style="stroke-width: 7.50; stroke: #D3D3D3;"></polyline><text x="24.00" y="66.72" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="149.64px" lengthadjust="spacingAndGlyphs">c(0, 13.2739734904165)</text><text transform="translate(-46.08,6.00) rotate(-90)" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="38.87px" lengthadjust="spacingAndGlyphs">c(1, 1)</text></g></svg>
#> </td>
#> <td style="text-align:right;"> -2.7372606 </td>
#> <td style="text-align:right;"> 0.2062126 </td>
#> <td style="text-align:right;"> <span style=" border-radius: 4px; padding-right: 4px; padding-left: 4px; background-color: #FFFFFF !important;">0</span> </td>
#> </tr>
#> <tr>
#> <td style="text-align:left;"> Age </td>
#> <td style="text-align:left;"> Age </td>
#> <td style="text-align:left;"> <svg xmlns="http://www.w3.org/2000/svg" xmlns:xlink="http://www.w3.org/1999/xlink" class="svglite" width="48.00pt" height="12.00pt" viewbox="0 0 48.00 12.00"><defs><style type="text/css">
#> .svglite line, .svglite polyline, .svglite polygon, .svglite path, .svglite rect, .svglite circle {
#> fill: none;
#> stroke: #000000;
#> stroke-linecap: round;
#> stroke-linejoin: round;
#> stroke-miterlimit: 10.00;
#> }
#> .svglite text {
#> white-space: pre;
#> }
#> </style></defs><rect width="100%" height="100%" style="stroke: none; fill: none;"></rect><defs><clippath id="cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw"><rect x="0.00" y="0.00" width="48.00" height="12.00"></rect></clippath></defs><g clip-path="url(#cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw)"><polyline points="3.42,6.00 19.12,6.00 " style="stroke-width: 7.50; stroke: #D3D3D3;"></polyline><text x="24.00" y="66.72" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="142.00px" lengthadjust="spacingAndGlyphs">c(0, 5.0617392607681)</text><text transform="translate(-46.08,6.00) rotate(-90)" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="38.87px" lengthadjust="spacingAndGlyphs">c(1, 1)</text></g></svg>
#> </td>
#> <td style="text-align:right;"> -0.0412313 </td>
#> <td style="text-align:right;"> 0.0081457 </td>
#> <td style="text-align:right;"> <span style=" border-radius: 4px; padding-right: 4px; padding-left: 4px; background-color: #FFFFFF !important;">0</span> </td>
#> </tr>
#> <tr>
#> <td style="text-align:left;"> Fare </td>
#> <td style="text-align:left;"> Fare </td>
#> <td style="text-align:left;"> <svg xmlns="http://www.w3.org/2000/svg" xmlns:xlink="http://www.w3.org/1999/xlink" class="svglite" width="48.00pt" height="12.00pt" viewbox="0 0 48.00 12.00"><defs><style type="text/css">
#> .svglite line, .svglite polyline, .svglite polygon, .svglite path, .svglite rect, .svglite circle {
#> fill: none;
#> stroke: #000000;
#> stroke-linecap: round;
#> stroke-linejoin: round;
#> stroke-miterlimit: 10.00;
#> }
#> .svglite text {
#> white-space: pre;
#> }
#> </style></defs><rect width="100%" height="100%" style="stroke: none; fill: none;"></rect><defs><clippath id="cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw"><rect x="0.00" y="0.00" width="48.00" height="12.00"></rect></clippath></defs><g clip-path="url(#cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw)"><polyline points="3.42,6.00 6.83,6.00 " style="stroke-width: 7.50; stroke: #D3D3D3;"></polyline><text x="24.00" y="66.72" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="149.64px" lengthadjust="spacingAndGlyphs">c(0, 1.09903343555086)</text><text transform="translate(-46.08,6.00) rotate(-90)" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="38.87px" lengthadjust="spacingAndGlyphs">c(1, 1)</text></g></svg>
#> </td>
#> <td style="text-align:right;"> 0.0029675 </td>
#> <td style="text-align:right;"> 0.0027001 </td>
#> <td style="text-align:right;"> <span style=" border-radius: 4px; padding-right: 4px; padding-left: 4px; background-color: #F08080 !important;">0.27175</span> </td>
#> </tr>
#> <tr>
#> <td style="text-align:left;"> Embarked </td>
#> <td style="text-align:left;"> C </td>
#> <td style="text-align:left;"> <svg xmlns="http://www.w3.org/2000/svg" xmlns:xlink="http://www.w3.org/1999/xlink" class="svglite" width="48.00pt" height="12.00pt" viewbox="0 0 48.00 12.00"><defs><style type="text/css">
#> .svglite line, .svglite polyline, .svglite polygon, .svglite path, .svglite rect, .svglite circle {
#> fill: none;
#> stroke: #000000;
#> stroke-linecap: round;
#> stroke-linejoin: round;
#> stroke-miterlimit: 10.00;
#> }
#> .svglite text {
#> white-space: pre;
#> }
#> </style></defs><rect width="100%" height="100%" style="stroke: none; fill: none;"></rect><defs><clippath id="cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw"><rect x="0.00" y="0.00" width="48.00" height="12.00"></rect></clippath></defs><g clip-path="url(#cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw)"><polyline points="3.42,6.00 3.42,6.00 " style="stroke-width: 7.50; stroke: #D3D3D3;"></polyline><text x="24.00" y="66.72" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="38.87px" lengthadjust="spacingAndGlyphs">c(0, 0)</text><text transform="translate(-46.08,6.00) rotate(-90)" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="38.87px" lengthadjust="spacingAndGlyphs">c(1, 1)</text></g></svg>
#> </td>
#> <td style="text-align:right;"> 0.0000000 </td>
#> <td style="text-align:right;"> 0.0000000 </td>
#> <td style="text-align:right;"> <span style=" border-radius: 4px; padding-right: 4px; padding-left: 4px; background-color: #FFFFFF !important;">NA</span> </td>
#> </tr>
#> <tr>
#> <td style="text-align:left;"> Embarked </td>
#> <td style="text-align:left;"> Q </td>
#> <td style="text-align:left;"> <svg xmlns="http://www.w3.org/2000/svg" xmlns:xlink="http://www.w3.org/1999/xlink" class="svglite" width="48.00pt" height="12.00pt" viewbox="0 0 48.00 12.00"><defs><style type="text/css">
#> .svglite line, .svglite polyline, .svglite polygon, .svglite path, .svglite rect, .svglite circle {
#> fill: none;
#> stroke: #000000;
#> stroke-linecap: round;
#> stroke-linejoin: round;
#> stroke-miterlimit: 10.00;
#> }
#> .svglite text {
#> white-space: pre;
#> }
#> </style></defs><rect width="100%" height="100%" style="stroke: none; fill: none;"></rect><defs><clippath id="cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw"><rect x="0.00" y="0.00" width="48.00" height="12.00"></rect></clippath></defs><g clip-path="url(#cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw)"><polyline points="3.42,6.00 4.05,6.00 " style="stroke-width: 7.50; stroke: #D3D3D3;"></polyline><text x="24.00" y="66.72" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="157.28px" lengthadjust="spacingAndGlyphs">c(0, 0.202849414475676)</text><text transform="translate(-46.08,6.00) rotate(-90)" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="38.87px" lengthadjust="spacingAndGlyphs">c(1, 1)</text></g></svg>
#> </td>
#> <td style="text-align:right;"> -0.0783606 </td>
#> <td style="text-align:right;"> 0.3862992 </td>
#> <td style="text-align:right;"> <span style=" border-radius: 4px; padding-right: 4px; padding-left: 4px; background-color: #F08080 !important;">0.83925</span> </td>
#> </tr>
#> <tr>
#> <td style="text-align:left;"> Embarked </td>
#> <td style="text-align:left;"> S </td>
#> <td style="text-align:left;"> <svg xmlns="http://www.w3.org/2000/svg" xmlns:xlink="http://www.w3.org/1999/xlink" class="svglite" width="48.00pt" height="12.00pt" viewbox="0 0 48.00 12.00"><defs><style type="text/css">
#> .svglite line, .svglite polyline, .svglite polygon, .svglite path, .svglite rect, .svglite circle {
#> fill: none;
#> stroke: #000000;
#> stroke-linecap: round;
#> stroke-linejoin: round;
#> stroke-miterlimit: 10.00;
#> }
#> .svglite text {
#> white-space: pre;
#> }
#> </style></defs><rect width="100%" height="100%" style="stroke: none; fill: none;"></rect><defs><clippath id="cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw"><rect x="0.00" y="0.00" width="48.00" height="12.00"></rect></clippath></defs><g clip-path="url(#cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw)"><polyline points="3.42,6.00 9.86,6.00 " style="stroke-width: 7.50; stroke: #D3D3D3;"></polyline><text x="24.00" y="66.72" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="149.64px" lengthadjust="spacingAndGlyphs">c(0, 2.07730489907646)</text><text transform="translate(-46.08,6.00) rotate(-90)" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="38.87px" lengthadjust="spacingAndGlyphs">c(1, 1)</text></g></svg>
#> </td>
#> <td style="text-align:right;"> -0.5118226 </td>
#> <td style="text-align:right;"> 0.2463878 </td>
#> <td style="text-align:right;"> <span style=" border-radius: 4px; padding-right: 4px; padding-left: 4px; background-color: #FFFFFF !important;">0.03777</span> </td>
#> </tr>
#> <tr>
#> <td style="text-align:left;"> SibSp </td>
#> <td style="text-align:left;"> SibSp </td>
#> <td style="text-align:left;"> <svg xmlns="http://www.w3.org/2000/svg" xmlns:xlink="http://www.w3.org/1999/xlink" class="svglite" width="48.00pt" height="12.00pt" viewbox="0 0 48.00 12.00"><defs><style type="text/css">
#> .svglite line, .svglite polyline, .svglite polygon, .svglite path, .svglite rect, .svglite circle {
#> fill: none;
#> stroke: #000000;
#> stroke-linecap: round;
#> stroke-linejoin: round;
#> stroke-miterlimit: 10.00;
#> }
#> .svglite text {
#> white-space: pre;
#> }
#> </style></defs><rect width="100%" height="100%" style="stroke: none; fill: none;"></rect><defs><clippath id="cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw"><rect x="0.00" y="0.00" width="48.00" height="12.00"></rect></clippath></defs><g clip-path="url(#cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw)"><polyline points="3.42,6.00 12.49,6.00 " style="stroke-width: 7.50; stroke: #D3D3D3;"></polyline><text x="24.00" y="66.72" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="149.64px" lengthadjust="spacingAndGlyphs">c(0, 2.92421389359779)</text><text transform="translate(-46.08,6.00) rotate(-90)" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="38.87px" lengthadjust="spacingAndGlyphs">c(1, 1)</text></g></svg>
#> </td>
#> <td style="text-align:right;"> -0.3251949 </td>
#> <td style="text-align:right;"> 0.1112076 </td>
#> <td style="text-align:right;"> <span style=" border-radius: 4px; padding-right: 4px; padding-left: 4px; background-color: #FFFFFF !important;">0.00345</span> </td>
#> </tr>
#> <tr>
#> <td style="text-align:left;"> Parch </td>
#> <td style="text-align:left;"> Parch </td>
#> <td style="text-align:left;"> <svg xmlns="http://www.w3.org/2000/svg" xmlns:xlink="http://www.w3.org/1999/xlink" class="svglite" width="48.00pt" height="12.00pt" viewbox="0 0 48.00 12.00"><defs><style type="text/css">
#> .svglite line, .svglite polyline, .svglite polygon, .svglite path, .svglite rect, .svglite circle {
#> fill: none;
#> stroke: #000000;
#> stroke-linecap: round;
#> stroke-linejoin: round;
#> stroke-miterlimit: 10.00;
#> }
#> .svglite text {
#> white-space: pre;
#> }
#> </style></defs><rect width="100%" height="100%" style="stroke: none; fill: none;"></rect><defs><clippath id="cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw"><rect x="0.00" y="0.00" width="48.00" height="12.00"></rect></clippath></defs><g clip-path="url(#cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw)"><polyline points="3.42,6.00 6.29,6.00 " style="stroke-width: 7.50; stroke: #D3D3D3;"></polyline><text x="24.00" y="66.72" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="157.28px" lengthadjust="spacingAndGlyphs">c(0, 0.923420665185219)</text><text transform="translate(-46.08,6.00) rotate(-90)" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="38.87px" lengthadjust="spacingAndGlyphs">c(1, 1)</text></g></svg>
#> </td>
#> <td style="text-align:right;"> -0.1115613 </td>
#> <td style="text-align:right;"> 0.1208131 </td>
#> <td style="text-align:right;"> <span style=" border-radius: 4px; padding-right: 4px; padding-left: 4px; background-color: #F08080 !important;">0.35579</span> </td>
#> </tr>
#> <tr>
#> <td style="text-align:left;"> Cabintype </td>
#> <td style="text-align:left;"> A </td>
#> <td style="text-align:left;"> <svg xmlns="http://www.w3.org/2000/svg" xmlns:xlink="http://www.w3.org/1999/xlink" class="svglite" width="48.00pt" height="12.00pt" viewbox="0 0 48.00 12.00"><defs><style type="text/css">
#> .svglite line, .svglite polyline, .svglite polygon, .svglite path, .svglite rect, .svglite circle {
#> fill: none;
#> stroke: #000000;
#> stroke-linecap: round;
#> stroke-linejoin: round;
#> stroke-miterlimit: 10.00;
#> }
#> .svglite text {
#> white-space: pre;
#> }
#> </style></defs><rect width="100%" height="100%" style="stroke: none; fill: none;"></rect><defs><clippath id="cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw"><rect x="0.00" y="0.00" width="48.00" height="12.00"></rect></clippath></defs><g clip-path="url(#cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw)"><polyline points="3.42,6.00 3.42,6.00 " style="stroke-width: 7.50; stroke: #D3D3D3;"></polyline><text x="24.00" y="66.72" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="38.87px" lengthadjust="spacingAndGlyphs">c(0, 0)</text><text transform="translate(-46.08,6.00) rotate(-90)" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="38.87px" lengthadjust="spacingAndGlyphs">c(1, 1)</text></g></svg>
#> </td>
#> <td style="text-align:right;"> 0.0000000 </td>
#> <td style="text-align:right;"> 0.0000000 </td>
#> <td style="text-align:right;"> <span style=" border-radius: 4px; padding-right: 4px; padding-left: 4px; background-color: #FFFFFF !important;">NA</span> </td>
#> </tr>
#> <tr>
#> <td style="text-align:left;"> Cabintype </td>
#> <td style="text-align:left;"> B </td>
#> <td style="text-align:left;"> <svg xmlns="http://www.w3.org/2000/svg" xmlns:xlink="http://www.w3.org/1999/xlink" class="svglite" width="48.00pt" height="12.00pt" viewbox="0 0 48.00 12.00"><defs><style type="text/css">
#> .svglite line, .svglite polyline, .svglite polygon, .svglite path, .svglite rect, .svglite circle {
#> fill: none;
#> stroke: #000000;
#> stroke-linecap: round;
#> stroke-linejoin: round;
#> stroke-miterlimit: 10.00;
#> }
#> .svglite text {
#> white-space: pre;
#> }
#> </style></defs><rect width="100%" height="100%" style="stroke: none; fill: none;"></rect><defs><clippath id="cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw"><rect x="0.00" y="0.00" width="48.00" height="12.00"></rect></clippath></defs><g clip-path="url(#cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw)"><polyline points="3.42,6.00 3.53,6.00 " style="stroke-width: 7.50; stroke: #D3D3D3;"></polyline><text x="24.00" y="66.72" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="164.92px" lengthadjust="spacingAndGlyphs">c(0, 0.0351335813748284)</text><text transform="translate(-46.08,6.00) rotate(-90)" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="38.87px" lengthadjust="spacingAndGlyphs">c(1, 1)</text></g></svg>
#> </td>
#> <td style="text-align:right;"> -0.0252365 </td>
#> <td style="text-align:right;"> 0.7183014 </td>
#> <td style="text-align:right;"> <span style=" border-radius: 4px; padding-right: 4px; padding-left: 4px; background-color: #F08080 !important;">0.97197</span> </td>
#> </tr>
#> <tr>
#> <td style="text-align:left;"> Cabintype </td>
#> <td style="text-align:left;"> C </td>
#> <td style="text-align:left;"> <svg xmlns="http://www.w3.org/2000/svg" xmlns:xlink="http://www.w3.org/1999/xlink" class="svglite" width="48.00pt" height="12.00pt" viewbox="0 0 48.00 12.00"><defs><style type="text/css">
#> .svglite line, .svglite polyline, .svglite polygon, .svglite path, .svglite rect, .svglite circle {
#> fill: none;
#> stroke: #000000;
#> stroke-linecap: round;
#> stroke-linejoin: round;
#> stroke-miterlimit: 10.00;
#> }
#> .svglite text {
#> white-space: pre;
#> }
#> </style></defs><rect width="100%" height="100%" style="stroke: none; fill: none;"></rect><defs><clippath id="cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw"><rect x="0.00" y="0.00" width="48.00" height="12.00"></rect></clippath></defs><g clip-path="url(#cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw)"><polyline points="3.42,6.00 5.77,6.00 " style="stroke-width: 7.50; stroke: #D3D3D3;"></polyline><text x="24.00" y="66.72" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="157.28px" lengthadjust="spacingAndGlyphs">c(0, 0.757567313946499)</text><text transform="translate(-46.08,6.00) rotate(-90)" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="38.87px" lengthadjust="spacingAndGlyphs">c(1, 1)</text></g></svg>
#> </td>
#> <td style="text-align:right;"> -0.5019796 </td>
#> <td style="text-align:right;"> 0.6626204 </td>
#> <td style="text-align:right;"> <span style=" border-radius: 4px; padding-right: 4px; padding-left: 4px; background-color: #F08080 !important;">0.44871</span> </td>
#> </tr>
#> <tr>
#> <td style="text-align:left;"> Cabintype </td>
#> <td style="text-align:left;"> D </td>
#> <td style="text-align:left;"> <svg xmlns="http://www.w3.org/2000/svg" xmlns:xlink="http://www.w3.org/1999/xlink" class="svglite" width="48.00pt" height="12.00pt" viewbox="0 0 48.00 12.00"><defs><style type="text/css">
#> .svglite line, .svglite polyline, .svglite polygon, .svglite path, .svglite rect, .svglite circle {
#> fill: none;
#> stroke: #000000;
#> stroke-linecap: round;
#> stroke-linejoin: round;
#> stroke-miterlimit: 10.00;
#> }
#> .svglite text {
#> white-space: pre;
#> }
#> </style></defs><rect width="100%" height="100%" style="stroke: none; fill: none;"></rect><defs><clippath id="cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw"><rect x="0.00" y="0.00" width="48.00" height="12.00"></rect></clippath></defs><g clip-path="url(#cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw)"><polyline points="3.42,6.00 5.44,6.00 " style="stroke-width: 7.50; stroke: #D3D3D3;"></polyline><text x="24.00" y="66.72" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="157.28px" lengthadjust="spacingAndGlyphs">c(0, 0.649346128379897)</text><text transform="translate(-46.08,6.00) rotate(-90)" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="38.87px" lengthadjust="spacingAndGlyphs">c(1, 1)</text></g></svg>
#> </td>
#> <td style="text-align:right;"> 0.4769107 </td>
#> <td style="text-align:right;"> 0.7344476 </td>
#> <td style="text-align:right;"> <span style=" border-radius: 4px; padding-right: 4px; padding-left: 4px; background-color: #F08080 !important;">0.51611</span> </td>
#> </tr>
#> <tr>
#> <td style="text-align:left;"> Cabintype </td>
#> <td style="text-align:left;"> E </td>
#> <td style="text-align:left;"> <svg xmlns="http://www.w3.org/2000/svg" xmlns:xlink="http://www.w3.org/1999/xlink" class="svglite" width="48.00pt" height="12.00pt" viewbox="0 0 48.00 12.00"><defs><style type="text/css">
#> .svglite line, .svglite polyline, .svglite polygon, .svglite path, .svglite rect, .svglite circle {
#> fill: none;
#> stroke: #000000;
#> stroke-linecap: round;
#> stroke-linejoin: round;
#> stroke-miterlimit: 10.00;
#> }
#> .svglite text {
#> white-space: pre;
#> }
#> </style></defs><rect width="100%" height="100%" style="stroke: none; fill: none;"></rect><defs><clippath id="cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw"><rect x="0.00" y="0.00" width="48.00" height="12.00"></rect></clippath></defs><g clip-path="url(#cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw)"><polyline points="3.42,6.00 7.58,6.00 " style="stroke-width: 7.50; stroke: #D3D3D3;"></polyline><text x="24.00" y="66.72" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="142.00px" lengthadjust="spacingAndGlyphs">c(0, 1.3389917872314)</text><text transform="translate(-46.08,6.00) rotate(-90)" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="38.87px" lengthadjust="spacingAndGlyphs">c(1, 1)</text></g></svg>
#> </td>
#> <td style="text-align:right;"> 0.9884183 </td>
#> <td style="text-align:right;"> 0.7381810 </td>
#> <td style="text-align:right;"> <span style=" border-radius: 4px; padding-right: 4px; padding-left: 4px; background-color: #F08080 !important;">0.18057</span> </td>
#> </tr>
#> <tr>
#> <td style="text-align:left;"> Cabintype </td>
#> <td style="text-align:left;"> F </td>
#> <td style="text-align:left;"> <svg xmlns="http://www.w3.org/2000/svg" xmlns:xlink="http://www.w3.org/1999/xlink" class="svglite" width="48.00pt" height="12.00pt" viewbox="0 0 48.00 12.00"><defs><style type="text/css">
#> .svglite line, .svglite polyline, .svglite polygon, .svglite path, .svglite rect, .svglite circle {
#> fill: none;
#> stroke: #000000;
#> stroke-linecap: round;
#> stroke-linejoin: round;
#> stroke-miterlimit: 10.00;
#> }
#> .svglite text {
#> white-space: pre;
#> }
#> </style></defs><rect width="100%" height="100%" style="stroke: none; fill: none;"></rect><defs><clippath id="cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw"><rect x="0.00" y="0.00" width="48.00" height="12.00"></rect></clippath></defs><g clip-path="url(#cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw)"><polyline points="3.42,6.00 4.36,6.00 " style="stroke-width: 7.50; stroke: #D3D3D3;"></polyline><text x="24.00" y="66.72" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="157.28px" lengthadjust="spacingAndGlyphs">c(0, 0.300798075499307)</text><text transform="translate(-46.08,6.00) rotate(-90)" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="38.87px" lengthadjust="spacingAndGlyphs">c(1, 1)</text></g></svg>
#> </td>
#> <td style="text-align:right;"> 0.2948757 </td>
#> <td style="text-align:right;"> 0.9803112 </td>
#> <td style="text-align:right;"> <span style=" border-radius: 4px; padding-right: 4px; padding-left: 4px; background-color: #F08080 !important;">0.76357</span> </td>
#> </tr>
#> <tr>
#> <td style="text-align:left;"> Cabintype </td>
#> <td style="text-align:left;"> G </td>
#> <td style="text-align:left;"> <svg xmlns="http://www.w3.org/2000/svg" xmlns:xlink="http://www.w3.org/1999/xlink" class="svglite" width="48.00pt" height="12.00pt" viewbox="0 0 48.00 12.00"><defs><style type="text/css">
#> .svglite line, .svglite polyline, .svglite polygon, .svglite path, .svglite rect, .svglite circle {
#> fill: none;
#> stroke: #000000;
#> stroke-linecap: round;
#> stroke-linejoin: round;
#> stroke-miterlimit: 10.00;
#> }
#> .svglite text {
#> white-space: pre;
#> }
#> </style></defs><rect width="100%" height="100%" style="stroke: none; fill: none;"></rect><defs><clippath id="cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw"><rect x="0.00" y="0.00" width="48.00" height="12.00"></rect></clippath></defs><g clip-path="url(#cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw)"><polyline points="3.42,6.00 7.31,6.00 " style="stroke-width: 7.50; stroke: #D3D3D3;"></polyline><text x="24.00" y="66.72" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="149.64px" lengthadjust="spacingAndGlyphs">c(0, 1.25434592564086)</text><text transform="translate(-46.08,6.00) rotate(-90)" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="38.87px" lengthadjust="spacingAndGlyphs">c(1, 1)</text></g></svg>
#> </td>
#> <td style="text-align:right;"> -1.5773340 </td>
#> <td style="text-align:right;"> 1.2574952 </td>
#> <td style="text-align:right;"> <span style=" border-radius: 4px; padding-right: 4px; padding-left: 4px; background-color: #F08080 !important;">0.20972</span> </td>
#> </tr>
#> <tr>
#> <td style="text-align:left;"> Cabintype </td>
#> <td style="text-align:left;"> Missing </td>
#> <td style="text-align:left;"> <svg xmlns="http://www.w3.org/2000/svg" xmlns:xlink="http://www.w3.org/1999/xlink" class="svglite" width="48.00pt" height="12.00pt" viewbox="0 0 48.00 12.00"><defs><style type="text/css">
#> .svglite line, .svglite polyline, .svglite polygon, .svglite path, .svglite rect, .svglite circle {
#> fill: none;
#> stroke: #000000;
#> stroke-linecap: round;
#> stroke-linejoin: round;
#> stroke-miterlimit: 10.00;
#> }
#> .svglite text {
#> white-space: pre;
#> }
#> </style></defs><rect width="100%" height="100%" style="stroke: none; fill: none;"></rect><defs><clippath id="cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw"><rect x="0.00" y="0.00" width="48.00" height="12.00"></rect></clippath></defs><g clip-path="url(#cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw)"><polyline points="3.42,6.00 7.63,6.00 " style="stroke-width: 7.50; stroke: #D3D3D3;"></polyline><text x="24.00" y="66.72" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="149.64px" lengthadjust="spacingAndGlyphs">c(0, 1.35752092721407)</text><text transform="translate(-46.08,6.00) rotate(-90)" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="38.87px" lengthadjust="spacingAndGlyphs">c(1, 1)</text></g></svg>
#> </td>
#> <td style="text-align:right;"> -0.9127259 </td>
#> <td style="text-align:right;"> 0.6723476 </td>
#> <td style="text-align:right;"> <span style=" border-radius: 4px; padding-right: 4px; padding-left: 4px; background-color: #F08080 !important;">0.17462</span> </td>
#> </tr>
#> <tr>
#> <td style="text-align:left;"> Cabintype </td>
#> <td style="text-align:left;"> T </td>
#> <td style="text-align:left;"> <svg xmlns="http://www.w3.org/2000/svg" xmlns:xlink="http://www.w3.org/1999/xlink" class="svglite" width="48.00pt" height="12.00pt" viewbox="0 0 48.00 12.00"><defs><style type="text/css">
#> .svglite line, .svglite polyline, .svglite polygon, .svglite path, .svglite rect, .svglite circle {
#> fill: none;
#> stroke: #000000;
#> stroke-linecap: round;
#> stroke-linejoin: round;
#> stroke-miterlimit: 10.00;
#> }
#> .svglite text {
#> white-space: pre;
#> }
#> </style></defs><rect width="100%" height="100%" style="stroke: none; fill: none;"></rect><defs><clippath id="cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw"><rect x="0.00" y="0.00" width="48.00" height="12.00"></rect></clippath></defs><g clip-path="url(#cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw)"><polyline points="3.42,6.00 3.50,6.00 " style="stroke-width: 7.50; stroke: #D3D3D3;"></polyline><text x="24.00" y="66.72" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="164.92px" lengthadjust="spacingAndGlyphs">c(0, 0.0241317350336744)</text><text transform="translate(-46.08,6.00) rotate(-90)" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="38.87px" lengthadjust="spacingAndGlyphs">c(1, 1)</text></g></svg>
#> </td>
#> <td style="text-align:right;"> -12.9204077 </td>
#> <td style="text-align:right;"> 535.4114701 </td>
#> <td style="text-align:right;"> <span style=" border-radius: 4px; padding-right: 4px; padding-left: 4px; background-color: #F08080 !important;">0.98075</span> </td>
#> </tr>
#> </tbody>
#> <tfoot><tr><td style="padding: 0; " colspan="100%">
#> <span style="font-style: italic;text-decoration: underline;">Goodness-of-Fit:</span> <sup></sup> AIC: 800.4 , Devience : 764.4 , Null Devience: 1182.8</td></tr></tfoot>
#> </table>
# A more complicated example with a spline and different importance method
survival_model3 <- stats::glm(Survived ~
Pclass +
Age_0_25 +
Age_25_50 +
Age_50_120 +
Sex:Fare_0_250 +
Sex:Fare_250_600 +
Embarked +
SibSp +
Parch +
Cabintype,
data = titanic,
family = binomial(link = 'logit'))
pretty_coefficients(survival_model3,
relativity_transform = 'exp(estimate)-1',
spline_seperator = '_',
vimethod = 'permute',
target = 'Survived',
metric = "roc_auc",
event_level = 'second',
pred_wrapper = predict.glm,
smaller_is_better = FALSE,
train = survival_model3$data, # need to supply training data for vip importance
reference_class = 0)
#> <table class="table" style="margin-left: auto; margin-right: auto;border-bottom: 0;">
#> <thead>
#> <tr>
#> <th style="text-align:left;"> Variable </th>
#> <th style="text-align:left;"> Level </th>
#> <th style="text-align:left;"> Importance </th>
#> <th style="text-align:right;"> Estimate </th>
#> <th style="text-align:right;"> Std.error </th>
#> <th style="text-align:right;"> Relativity </th>
#> <th style="text-align:right;"> P.Value </th>
#> </tr>
#> </thead>
#> <tbody>
#> <tr>
#> <td style="text-align:left;"> (Intercept) </td>
#> <td style="text-align:left;"> (Intercept) </td>
#> <td style="text-align:left;"> <svg xmlns="http://www.w3.org/2000/svg" xmlns:xlink="http://www.w3.org/1999/xlink" class="svglite" width="48.00pt" height="12.00pt" viewbox="0 0 48.00 12.00"><defs><style type="text/css">
#> .svglite line, .svglite polyline, .svglite polygon, .svglite path, .svglite rect, .svglite circle {
#> fill: none;
#> stroke: #000000;
#> stroke-linecap: round;
#> stroke-linejoin: round;
#> stroke-miterlimit: 10.00;
#> }
#> .svglite text {
#> white-space: pre;
#> }
#> </style></defs><rect width="100%" height="100%" style="stroke: none; fill: none;"></rect><defs><clippath id="cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw"><rect x="0.00" y="0.00" width="48.00" height="12.00"></rect></clippath></defs><g clip-path="url(#cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw)"><polyline points="3.42,6.00 3.42,6.00 " style="stroke-width: 7.50; stroke: #D3D3D3;"></polyline><text x="24.00" y="66.72" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="38.87px" lengthadjust="spacingAndGlyphs">c(0, 0)</text><text transform="translate(-46.08,6.00) rotate(-90)" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="38.87px" lengthadjust="spacingAndGlyphs">c(1, 1)</text></g></svg>
#> </td>
#> <td style="text-align:right;"> 2.9939893 </td>
#> <td style="text-align:right;"> 0.7390224 </td>
#> <td style="text-align:right;"> 18.9651706 </td>
#> <td style="text-align:right;"> <span style=" border-radius: 4px; padding-right: 4px; padding-left: 4px; background-color: #FFFFFF !important;">5e-05</span> </td>
#> </tr>
#> <tr>
#> <td style="text-align:left;"> Pclass </td>
#> <td style="text-align:left;"> 1 </td>
#> <td style="text-align:left;"> <svg xmlns="http://www.w3.org/2000/svg" xmlns:xlink="http://www.w3.org/1999/xlink" class="svglite" width="48.00pt" height="12.00pt" viewbox="0 0 48.00 12.00"><defs><style type="text/css">
#> .svglite line, .svglite polyline, .svglite polygon, .svglite path, .svglite rect, .svglite circle {
#> fill: none;
#> stroke: #000000;
#> stroke-linecap: round;
#> stroke-linejoin: round;
#> stroke-miterlimit: 10.00;
#> }
#> .svglite text {
#> white-space: pre;
#> }
#> </style></defs><rect width="100%" height="100%" style="stroke: none; fill: none;"></rect><defs><clippath id="cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw"><rect x="0.00" y="0.00" width="48.00" height="12.00"></rect></clippath></defs><g clip-path="url(#cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw)"><polyline points="3.42,6.00 7.79,6.00 " style="stroke-width: 7.50; stroke: #D3D3D3;"></polyline><text x="24.00" y="66.72" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="164.92px" lengthadjust="spacingAndGlyphs">c(0, 0.0164309439622844)</text><text transform="translate(-46.08,6.00) rotate(-90)" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="38.87px" lengthadjust="spacingAndGlyphs">c(1, 1)</text></g></svg>
#> </td>
#> <td style="text-align:right;"> 0.0000000 </td>
#> <td style="text-align:right;"> 0.0000000 </td>
#> <td style="text-align:right;"> 0.0000000 </td>
#> <td style="text-align:right;"> <span style=" border-radius: 4px; padding-right: 4px; padding-left: 4px; background-color: #FFFFFF !important;">NA</span> </td>
#> </tr>
#> <tr>
#> <td style="text-align:left;"> Pclass </td>
#> <td style="text-align:left;"> 2 </td>
#> <td style="text-align:left;"> <svg xmlns="http://www.w3.org/2000/svg" xmlns:xlink="http://www.w3.org/1999/xlink" class="svglite" width="48.00pt" height="12.00pt" viewbox="0 0 48.00 12.00"><defs><style type="text/css">
#> .svglite line, .svglite polyline, .svglite polygon, .svglite path, .svglite rect, .svglite circle {
#> fill: none;
#> stroke: #000000;
#> stroke-linecap: round;
#> stroke-linejoin: round;
#> stroke-miterlimit: 10.00;
#> }
#> .svglite text {
#> white-space: pre;
#> }
#> </style></defs><rect width="100%" height="100%" style="stroke: none; fill: none;"></rect><defs><clippath id="cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw"><rect x="0.00" y="0.00" width="48.00" height="12.00"></rect></clippath></defs><g clip-path="url(#cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw)"><polyline points="3.42,6.00 7.79,6.00 " style="stroke-width: 7.50; stroke: #D3D3D3;"></polyline><text x="24.00" y="66.72" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="164.92px" lengthadjust="spacingAndGlyphs">c(0, 0.0164309439622844)</text><text transform="translate(-46.08,6.00) rotate(-90)" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="38.87px" lengthadjust="spacingAndGlyphs">c(1, 1)</text></g></svg>
#> </td>
#> <td style="text-align:right;"> 0.1968365 </td>
#> <td style="text-align:right;"> 0.4640097 </td>
#> <td style="text-align:right;"> 0.2175450 </td>
#> <td style="text-align:right;"> <span style=" border-radius: 4px; padding-right: 4px; padding-left: 4px; background-color: #F08080 !important;">0.67141</span> </td>
#> </tr>
#> <tr>
#> <td style="text-align:left;"> Pclass </td>
#> <td style="text-align:left;"> 3 </td>
#> <td style="text-align:left;"> <svg xmlns="http://www.w3.org/2000/svg" xmlns:xlink="http://www.w3.org/1999/xlink" class="svglite" width="48.00pt" height="12.00pt" viewbox="0 0 48.00 12.00"><defs><style type="text/css">
#> .svglite line, .svglite polyline, .svglite polygon, .svglite path, .svglite rect, .svglite circle {
#> fill: none;
#> stroke: #000000;
#> stroke-linecap: round;
#> stroke-linejoin: round;
#> stroke-miterlimit: 10.00;
#> }
#> .svglite text {
#> white-space: pre;
#> }
#> </style></defs><rect width="100%" height="100%" style="stroke: none; fill: none;"></rect><defs><clippath id="cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw"><rect x="0.00" y="0.00" width="48.00" height="12.00"></rect></clippath></defs><g clip-path="url(#cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw)"><polyline points="3.42,6.00 7.79,6.00 " style="stroke-width: 7.50; stroke: #D3D3D3;"></polyline><text x="24.00" y="66.72" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="164.92px" lengthadjust="spacingAndGlyphs">c(0, 0.0164309439622844)</text><text transform="translate(-46.08,6.00) rotate(-90)" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="38.87px" lengthadjust="spacingAndGlyphs">c(1, 1)</text></g></svg>
#> </td>
#> <td style="text-align:right;"> -0.8373909 </td>
#> <td style="text-align:right;"> 0.4732294 </td>
#> <td style="text-align:right;"> -0.5671616 </td>
#> <td style="text-align:right;"> <span style=" border-radius: 4px; padding-right: 4px; padding-left: 4px; background-color: #F08080 !important;">0.07681</span> </td>
#> </tr>
#> <tr>
#> <td style="text-align:left;"> Age </td>
#> <td style="text-align:left;"> Age_0_25 </td>
#> <td style="text-align:left;"> <svg xmlns="http://www.w3.org/2000/svg" xmlns:xlink="http://www.w3.org/1999/xlink" class="svglite" width="48.00pt" height="12.00pt" viewbox="0 0 48.00 12.00"><defs><style type="text/css">
#> .svglite line, .svglite polyline, .svglite polygon, .svglite path, .svglite rect, .svglite circle {
#> fill: none;
#> stroke: #000000;
#> stroke-linecap: round;
#> stroke-linejoin: round;
#> stroke-miterlimit: 10.00;
#> }
#> .svglite text {
#> white-space: pre;
#> }
#> </style></defs><rect width="100%" height="100%" style="stroke: none; fill: none;"></rect><defs><clippath id="cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw"><rect x="0.00" y="0.00" width="48.00" height="12.00"></rect></clippath></defs><g clip-path="url(#cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw)"><polyline points="3.42,6.00 13.61,6.00 " style="stroke-width: 7.50; stroke: #D3D3D3;"></polyline><text x="24.00" y="66.72" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="164.92px" lengthadjust="spacingAndGlyphs">c(0, 0.0383665488053145)</text><text transform="translate(-46.08,6.00) rotate(-90)" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="38.87px" lengthadjust="spacingAndGlyphs">c(1, 1)</text></g></svg>
#> </td>
#> <td style="text-align:right;"> -0.0932714 </td>
#> <td style="text-align:right;"> 0.0184680 </td>
#> <td style="text-align:right;"> -0.0890537 </td>
#> <td style="text-align:right;"> <span style=" border-radius: 4px; padding-right: 4px; padding-left: 4px; background-color: #FFFFFF !important;">0</span> </td>
#> </tr>
#> <tr>
#> <td style="text-align:left;"> Age </td>
#> <td style="text-align:left;"> Age_25_50 </td>
#> <td style="text-align:left;"> <svg xmlns="http://www.w3.org/2000/svg" xmlns:xlink="http://www.w3.org/1999/xlink" class="svglite" width="48.00pt" height="12.00pt" viewbox="0 0 48.00 12.00"><defs><style type="text/css">
#> .svglite line, .svglite polyline, .svglite polygon, .svglite path, .svglite rect, .svglite circle {
#> fill: none;
#> stroke: #000000;
#> stroke-linecap: round;
#> stroke-linejoin: round;
#> stroke-miterlimit: 10.00;
#> }
#> .svglite text {
#> white-space: pre;
#> }
#> </style></defs><rect width="100%" height="100%" style="stroke: none; fill: none;"></rect><defs><clippath id="cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw"><rect x="0.00" y="0.00" width="48.00" height="12.00"></rect></clippath></defs><g clip-path="url(#cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw)"><polyline points="3.42,6.00 4.11,6.00 " style="stroke-width: 7.50; stroke: #D3D3D3;"></polyline><text x="24.00" y="66.72" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="172.56px" lengthadjust="spacingAndGlyphs">c(0, 0.00257152041144326)</text><text transform="translate(-46.08,6.00) rotate(-90)" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="38.87px" lengthadjust="spacingAndGlyphs">c(1, 1)</text></g></svg>
#> </td>
#> <td style="text-align:right;"> -0.0167502 </td>
#> <td style="text-align:right;"> 0.0152050 </td>
#> <td style="text-align:right;"> -0.0166107 </td>
#> <td style="text-align:right;"> <span style=" border-radius: 4px; padding-right: 4px; padding-left: 4px; background-color: #F08080 !important;">0.27063</span> </td>
#> </tr>
#> <tr>
#> <td style="text-align:left;"> Age </td>
#> <td style="text-align:left;"> Age_50_120 </td>
#> <td style="text-align:left;"> <svg xmlns="http://www.w3.org/2000/svg" xmlns:xlink="http://www.w3.org/1999/xlink" class="svglite" width="48.00pt" height="12.00pt" viewbox="0 0 48.00 12.00"><defs><style type="text/css">
#> .svglite line, .svglite polyline, .svglite polygon, .svglite path, .svglite rect, .svglite circle {
#> fill: none;
#> stroke: #000000;
#> stroke-linecap: round;
#> stroke-linejoin: round;
#> stroke-miterlimit: 10.00;
#> }
#> .svglite text {
#> white-space: pre;
#> }
#> </style></defs><rect width="100%" height="100%" style="stroke: none; fill: none;"></rect><defs><clippath id="cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw"><rect x="0.00" y="0.00" width="48.00" height="12.00"></rect></clippath></defs><g clip-path="url(#cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw)"><polyline points="3.42,6.00 3.81,6.00 " style="stroke-width: 7.50; stroke: #D3D3D3;"></polyline><text x="24.00" y="66.72" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="172.56px" lengthadjust="spacingAndGlyphs">c(0, 0.00145451623272252)</text><text transform="translate(-46.08,6.00) rotate(-90)" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="38.87px" lengthadjust="spacingAndGlyphs">c(1, 1)</text></g></svg>
#> </td>
#> <td style="text-align:right;"> -0.0500033 </td>
#> <td style="text-align:right;"> 0.0420905 </td>
#> <td style="text-align:right;"> -0.0487738 </td>
#> <td style="text-align:right;"> <span style=" border-radius: 4px; padding-right: 4px; padding-left: 4px; background-color: #F08080 !important;">0.23484</span> </td>
#> </tr>
#> <tr>
#> <td style="text-align:left;"> Embarked </td>
#> <td style="text-align:left;"> C </td>
#> <td style="text-align:left;"> <svg xmlns="http://www.w3.org/2000/svg" xmlns:xlink="http://www.w3.org/1999/xlink" class="svglite" width="48.00pt" height="12.00pt" viewbox="0 0 48.00 12.00"><defs><style type="text/css">
#> .svglite line, .svglite polyline, .svglite polygon, .svglite path, .svglite rect, .svglite circle {
#> fill: none;
#> stroke: #000000;
#> stroke-linecap: round;
#> stroke-linejoin: round;
#> stroke-miterlimit: 10.00;
#> }
#> .svglite text {
#> white-space: pre;
#> }
#> </style></defs><rect width="100%" height="100%" style="stroke: none; fill: none;"></rect><defs><clippath id="cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw"><rect x="0.00" y="0.00" width="48.00" height="12.00"></rect></clippath></defs><g clip-path="url(#cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw)"><polyline points="3.42,6.00 5.84,6.00 " style="stroke-width: 7.50; stroke: #D3D3D3;"></polyline><text x="24.00" y="66.72" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="172.56px" lengthadjust="spacingAndGlyphs">c(0, 0.00908510635384607)</text><text transform="translate(-46.08,6.00) rotate(-90)" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="38.87px" lengthadjust="spacingAndGlyphs">c(1, 1)</text></g></svg>
#> </td>
#> <td style="text-align:right;"> 0.0000000 </td>
#> <td style="text-align:right;"> 0.0000000 </td>
#> <td style="text-align:right;"> 0.0000000 </td>
#> <td style="text-align:right;"> <span style=" border-radius: 4px; padding-right: 4px; padding-left: 4px; background-color: #FFFFFF !important;">NA</span> </td>
#> </tr>
#> <tr>
#> <td style="text-align:left;"> Embarked </td>
#> <td style="text-align:left;"> Q </td>
#> <td style="text-align:left;"> <svg xmlns="http://www.w3.org/2000/svg" xmlns:xlink="http://www.w3.org/1999/xlink" class="svglite" width="48.00pt" height="12.00pt" viewbox="0 0 48.00 12.00"><defs><style type="text/css">
#> .svglite line, .svglite polyline, .svglite polygon, .svglite path, .svglite rect, .svglite circle {
#> fill: none;
#> stroke: #000000;
#> stroke-linecap: round;
#> stroke-linejoin: round;
#> stroke-miterlimit: 10.00;
#> }
#> .svglite text {
#> white-space: pre;
#> }
#> </style></defs><rect width="100%" height="100%" style="stroke: none; fill: none;"></rect><defs><clippath id="cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw"><rect x="0.00" y="0.00" width="48.00" height="12.00"></rect></clippath></defs><g clip-path="url(#cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw)"><polyline points="3.42,6.00 5.84,6.00 " style="stroke-width: 7.50; stroke: #D3D3D3;"></polyline><text x="24.00" y="66.72" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="172.56px" lengthadjust="spacingAndGlyphs">c(0, 0.00908510635384607)</text><text transform="translate(-46.08,6.00) rotate(-90)" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="38.87px" lengthadjust="spacingAndGlyphs">c(1, 1)</text></g></svg>
#> </td>
#> <td style="text-align:right;"> 0.4151234 </td>
#> <td style="text-align:right;"> 0.3398956 </td>
#> <td style="text-align:right;"> 0.5145577 </td>
#> <td style="text-align:right;"> <span style=" border-radius: 4px; padding-right: 4px; padding-left: 4px; background-color: #F08080 !important;">0.22196</span> </td>
#> </tr>
#> <tr>
#> <td style="text-align:left;"> Embarked </td>
#> <td style="text-align:left;"> S </td>
#> <td style="text-align:left;"> <svg xmlns="http://www.w3.org/2000/svg" xmlns:xlink="http://www.w3.org/1999/xlink" class="svglite" width="48.00pt" height="12.00pt" viewbox="0 0 48.00 12.00"><defs><style type="text/css">
#> .svglite line, .svglite polyline, .svglite polygon, .svglite path, .svglite rect, .svglite circle {
#> fill: none;
#> stroke: #000000;
#> stroke-linecap: round;
#> stroke-linejoin: round;
#> stroke-miterlimit: 10.00;
#> }
#> .svglite text {
#> white-space: pre;
#> }
#> </style></defs><rect width="100%" height="100%" style="stroke: none; fill: none;"></rect><defs><clippath id="cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw"><rect x="0.00" y="0.00" width="48.00" height="12.00"></rect></clippath></defs><g clip-path="url(#cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw)"><polyline points="3.42,6.00 5.84,6.00 " style="stroke-width: 7.50; stroke: #D3D3D3;"></polyline><text x="24.00" y="66.72" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="172.56px" lengthadjust="spacingAndGlyphs">c(0, 0.00908510635384607)</text><text transform="translate(-46.08,6.00) rotate(-90)" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="38.87px" lengthadjust="spacingAndGlyphs">c(1, 1)</text></g></svg>
#> </td>
#> <td style="text-align:right;"> -0.5942634 </td>
#> <td style="text-align:right;"> 0.2398745 </td>
#> <td style="text-align:right;"> -0.4480310 </td>
#> <td style="text-align:right;"> <span style=" border-radius: 4px; padding-right: 4px; padding-left: 4px; background-color: #FFFFFF !important;">0.01323</span> </td>
#> </tr>
#> <tr>
#> <td style="text-align:left;"> SibSp </td>
#> <td style="text-align:left;"> SibSp </td>
#> <td style="text-align:left;"> <svg xmlns="http://www.w3.org/2000/svg" xmlns:xlink="http://www.w3.org/1999/xlink" class="svglite" width="48.00pt" height="12.00pt" viewbox="0 0 48.00 12.00"><defs><style type="text/css">
#> .svglite line, .svglite polyline, .svglite polygon, .svglite path, .svglite rect, .svglite circle {
#> fill: none;
#> stroke: #000000;
#> stroke-linecap: round;
#> stroke-linejoin: round;
#> stroke-miterlimit: 10.00;
#> }
#> .svglite text {
#> white-space: pre;
#> }
#> </style></defs><rect width="100%" height="100%" style="stroke: none; fill: none;"></rect><defs><clippath id="cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw"><rect x="0.00" y="0.00" width="48.00" height="12.00"></rect></clippath></defs><g clip-path="url(#cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw)"><polyline points="3.42,6.00 8.14,6.00 " style="stroke-width: 7.50; stroke: #D3D3D3;"></polyline><text x="24.00" y="66.72" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="164.92px" lengthadjust="spacingAndGlyphs">c(0, 0.0177622415086254)</text><text transform="translate(-46.08,6.00) rotate(-90)" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="38.87px" lengthadjust="spacingAndGlyphs">c(1, 1)</text></g></svg>
#> </td>
#> <td style="text-align:right;"> -0.5080500 </td>
#> <td style="text-align:right;"> 0.1178176 </td>
#> <td style="text-align:right;"> -0.3983323 </td>
#> <td style="text-align:right;"> <span style=" border-radius: 4px; padding-right: 4px; padding-left: 4px; background-color: #FFFFFF !important;">2e-05</span> </td>
#> </tr>
#> <tr>
#> <td style="text-align:left;"> Parch </td>
#> <td style="text-align:left;"> Parch </td>
#> <td style="text-align:left;"> <svg xmlns="http://www.w3.org/2000/svg" xmlns:xlink="http://www.w3.org/1999/xlink" class="svglite" width="48.00pt" height="12.00pt" viewbox="0 0 48.00 12.00"><defs><style type="text/css">
#> .svglite line, .svglite polyline, .svglite polygon, .svglite path, .svglite rect, .svglite circle {
#> fill: none;
#> stroke: #000000;
#> stroke-linecap: round;
#> stroke-linejoin: round;
#> stroke-miterlimit: 10.00;
#> }
#> .svglite text {
#> white-space: pre;
#> }
#> </style></defs><rect width="100%" height="100%" style="stroke: none; fill: none;"></rect><defs><clippath id="cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw"><rect x="0.00" y="0.00" width="48.00" height="12.00"></rect></clippath></defs><g clip-path="url(#cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw)"><polyline points="3.42,6.00 4.48,6.00 " style="stroke-width: 7.50; stroke: #D3D3D3;"></polyline><text x="24.00" y="66.72" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="172.56px" lengthadjust="spacingAndGlyphs">c(0, 0.00398317797064185)</text><text transform="translate(-46.08,6.00) rotate(-90)" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="38.87px" lengthadjust="spacingAndGlyphs">c(1, 1)</text></g></svg>
#> </td>
#> <td style="text-align:right;"> -0.1604124 </td>
#> <td style="text-align:right;"> 0.1369624 </td>
#> <td style="text-align:right;"> -0.1482075 </td>
#> <td style="text-align:right;"> <span style=" border-radius: 4px; padding-right: 4px; padding-left: 4px; background-color: #F08080 !important;">0.24151</span> </td>
#> </tr>
#> <tr>
#> <td style="text-align:left;"> Cabintype </td>
#> <td style="text-align:left;"> A </td>
#> <td style="text-align:left;"> <svg xmlns="http://www.w3.org/2000/svg" xmlns:xlink="http://www.w3.org/1999/xlink" class="svglite" width="48.00pt" height="12.00pt" viewbox="0 0 48.00 12.00"><defs><style type="text/css">
#> .svglite line, .svglite polyline, .svglite polygon, .svglite path, .svglite rect, .svglite circle {
#> fill: none;
#> stroke: #000000;
#> stroke-linecap: round;
#> stroke-linejoin: round;
#> stroke-miterlimit: 10.00;
#> }
#> .svglite text {
#> white-space: pre;
#> }
#> </style></defs><rect width="100%" height="100%" style="stroke: none; fill: none;"></rect><defs><clippath id="cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw"><rect x="0.00" y="0.00" width="48.00" height="12.00"></rect></clippath></defs><g clip-path="url(#cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw)"><polyline points="3.42,6.00 11.40,6.00 " style="stroke-width: 7.50; stroke: #D3D3D3;"></polyline><text x="24.00" y="66.72" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="164.92px" lengthadjust="spacingAndGlyphs">c(0, 0.0300225008036001)</text><text transform="translate(-46.08,6.00) rotate(-90)" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="38.87px" lengthadjust="spacingAndGlyphs">c(1, 1)</text></g></svg>
#> </td>
#> <td style="text-align:right;"> 0.0000000 </td>
#> <td style="text-align:right;"> 0.0000000 </td>
#> <td style="text-align:right;"> 0.0000000 </td>
#> <td style="text-align:right;"> <span style=" border-radius: 4px; padding-right: 4px; padding-left: 4px; background-color: #FFFFFF !important;">NA</span> </td>
#> </tr>
#> <tr>
#> <td style="text-align:left;"> Cabintype </td>
#> <td style="text-align:left;"> B </td>
#> <td style="text-align:left;"> <svg xmlns="http://www.w3.org/2000/svg" xmlns:xlink="http://www.w3.org/1999/xlink" class="svglite" width="48.00pt" height="12.00pt" viewbox="0 0 48.00 12.00"><defs><style type="text/css">
#> .svglite line, .svglite polyline, .svglite polygon, .svglite path, .svglite rect, .svglite circle {
#> fill: none;
#> stroke: #000000;
#> stroke-linecap: round;
#> stroke-linejoin: round;
#> stroke-miterlimit: 10.00;
#> }
#> .svglite text {
#> white-space: pre;
#> }
#> </style></defs><rect width="100%" height="100%" style="stroke: none; fill: none;"></rect><defs><clippath id="cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw"><rect x="0.00" y="0.00" width="48.00" height="12.00"></rect></clippath></defs><g clip-path="url(#cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw)"><polyline points="3.42,6.00 11.40,6.00 " style="stroke-width: 7.50; stroke: #D3D3D3;"></polyline><text x="24.00" y="66.72" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="164.92px" lengthadjust="spacingAndGlyphs">c(0, 0.0300225008036001)</text><text transform="translate(-46.08,6.00) rotate(-90)" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="38.87px" lengthadjust="spacingAndGlyphs">c(1, 1)</text></g></svg>
#> </td>
#> <td style="text-align:right;"> -0.0566639 </td>
#> <td style="text-align:right;"> 0.7580796 </td>
#> <td style="text-align:right;"> -0.0550884 </td>
#> <td style="text-align:right;"> <span style=" border-radius: 4px; padding-right: 4px; padding-left: 4px; background-color: #F08080 !important;">0.94042</span> </td>
#> </tr>
#> <tr>
#> <td style="text-align:left;"> Cabintype </td>
#> <td style="text-align:left;"> C </td>
#> <td style="text-align:left;"> <svg xmlns="http://www.w3.org/2000/svg" xmlns:xlink="http://www.w3.org/1999/xlink" class="svglite" width="48.00pt" height="12.00pt" viewbox="0 0 48.00 12.00"><defs><style type="text/css">
#> .svglite line, .svglite polyline, .svglite polygon, .svglite path, .svglite rect, .svglite circle {
#> fill: none;
#> stroke: #000000;
#> stroke-linecap: round;
#> stroke-linejoin: round;
#> stroke-miterlimit: 10.00;
#> }
#> .svglite text {
#> white-space: pre;
#> }
#> </style></defs><rect width="100%" height="100%" style="stroke: none; fill: none;"></rect><defs><clippath id="cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw"><rect x="0.00" y="0.00" width="48.00" height="12.00"></rect></clippath></defs><g clip-path="url(#cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw)"><polyline points="3.42,6.00 11.40,6.00 " style="stroke-width: 7.50; stroke: #D3D3D3;"></polyline><text x="24.00" y="66.72" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="164.92px" lengthadjust="spacingAndGlyphs">c(0, 0.0300225008036001)</text><text transform="translate(-46.08,6.00) rotate(-90)" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="38.87px" lengthadjust="spacingAndGlyphs">c(1, 1)</text></g></svg>
#> </td>
#> <td style="text-align:right;"> -0.4178996 </td>
#> <td style="text-align:right;"> 0.7019732 </td>
#> <td style="text-align:right;"> -0.3415717 </td>
#> <td style="text-align:right;"> <span style=" border-radius: 4px; padding-right: 4px; padding-left: 4px; background-color: #F08080 !important;">0.55163</span> </td>
#> </tr>
#> <tr>
#> <td style="text-align:left;"> Cabintype </td>
#> <td style="text-align:left;"> D </td>
#> <td style="text-align:left;"> <svg xmlns="http://www.w3.org/2000/svg" xmlns:xlink="http://www.w3.org/1999/xlink" class="svglite" width="48.00pt" height="12.00pt" viewbox="0 0 48.00 12.00"><defs><style type="text/css">
#> .svglite line, .svglite polyline, .svglite polygon, .svglite path, .svglite rect, .svglite circle {
#> fill: none;
#> stroke: #000000;
#> stroke-linecap: round;
#> stroke-linejoin: round;
#> stroke-miterlimit: 10.00;
#> }
#> .svglite text {
#> white-space: pre;
#> }
#> </style></defs><rect width="100%" height="100%" style="stroke: none; fill: none;"></rect><defs><clippath id="cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw"><rect x="0.00" y="0.00" width="48.00" height="12.00"></rect></clippath></defs><g clip-path="url(#cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw)"><polyline points="3.42,6.00 11.40,6.00 " style="stroke-width: 7.50; stroke: #D3D3D3;"></polyline><text x="24.00" y="66.72" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="164.92px" lengthadjust="spacingAndGlyphs">c(0, 0.0300225008036001)</text><text transform="translate(-46.08,6.00) rotate(-90)" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="38.87px" lengthadjust="spacingAndGlyphs">c(1, 1)</text></g></svg>
#> </td>
#> <td style="text-align:right;"> 0.4916346 </td>
#> <td style="text-align:right;"> 0.7572964 </td>
#> <td style="text-align:right;"> 0.6349866 </td>
#> <td style="text-align:right;"> <span style=" border-radius: 4px; padding-right: 4px; padding-left: 4px; background-color: #F08080 !important;">0.51621</span> </td>
#> </tr>
#> <tr>
#> <td style="text-align:left;"> Cabintype </td>
#> <td style="text-align:left;"> E </td>
#> <td style="text-align:left;"> <svg xmlns="http://www.w3.org/2000/svg" xmlns:xlink="http://www.w3.org/1999/xlink" class="svglite" width="48.00pt" height="12.00pt" viewbox="0 0 48.00 12.00"><defs><style type="text/css">
#> .svglite line, .svglite polyline, .svglite polygon, .svglite path, .svglite rect, .svglite circle {
#> fill: none;
#> stroke: #000000;
#> stroke-linecap: round;
#> stroke-linejoin: round;
#> stroke-miterlimit: 10.00;
#> }
#> .svglite text {
#> white-space: pre;
#> }
#> </style></defs><rect width="100%" height="100%" style="stroke: none; fill: none;"></rect><defs><clippath id="cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw"><rect x="0.00" y="0.00" width="48.00" height="12.00"></rect></clippath></defs><g clip-path="url(#cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw)"><polyline points="3.42,6.00 11.40,6.00 " style="stroke-width: 7.50; stroke: #D3D3D3;"></polyline><text x="24.00" y="66.72" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="164.92px" lengthadjust="spacingAndGlyphs">c(0, 0.0300225008036001)</text><text transform="translate(-46.08,6.00) rotate(-90)" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="38.87px" lengthadjust="spacingAndGlyphs">c(1, 1)</text></g></svg>
#> </td>
#> <td style="text-align:right;"> 0.9706608 </td>
#> <td style="text-align:right;"> 0.7440859 </td>
#> <td style="text-align:right;"> 1.6396882 </td>
#> <td style="text-align:right;"> <span style=" border-radius: 4px; padding-right: 4px; padding-left: 4px; background-color: #F08080 !important;">0.19206</span> </td>
#> </tr>
#> <tr>
#> <td style="text-align:left;"> Cabintype </td>
#> <td style="text-align:left;"> F </td>
#> <td style="text-align:left;"> <svg xmlns="http://www.w3.org/2000/svg" xmlns:xlink="http://www.w3.org/1999/xlink" class="svglite" width="48.00pt" height="12.00pt" viewbox="0 0 48.00 12.00"><defs><style type="text/css">
#> .svglite line, .svglite polyline, .svglite polygon, .svglite path, .svglite rect, .svglite circle {
#> fill: none;
#> stroke: #000000;
#> stroke-linecap: round;
#> stroke-linejoin: round;
#> stroke-miterlimit: 10.00;
#> }
#> .svglite text {
#> white-space: pre;
#> }
#> </style></defs><rect width="100%" height="100%" style="stroke: none; fill: none;"></rect><defs><clippath id="cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw"><rect x="0.00" y="0.00" width="48.00" height="12.00"></rect></clippath></defs><g clip-path="url(#cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw)"><polyline points="3.42,6.00 11.40,6.00 " style="stroke-width: 7.50; stroke: #D3D3D3;"></polyline><text x="24.00" y="66.72" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="164.92px" lengthadjust="spacingAndGlyphs">c(0, 0.0300225008036001)</text><text transform="translate(-46.08,6.00) rotate(-90)" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="38.87px" lengthadjust="spacingAndGlyphs">c(1, 1)</text></g></svg>
#> </td>
#> <td style="text-align:right;"> -0.0772653 </td>
#> <td style="text-align:right;"> 0.9482161 </td>
#> <td style="text-align:right;"> -0.0743558 </td>
#> <td style="text-align:right;"> <span style=" border-radius: 4px; padding-right: 4px; padding-left: 4px; background-color: #F08080 !important;">0.93506</span> </td>
#> </tr>
#> <tr>
#> <td style="text-align:left;"> Cabintype </td>
#> <td style="text-align:left;"> G </td>
#> <td style="text-align:left;"> <svg xmlns="http://www.w3.org/2000/svg" xmlns:xlink="http://www.w3.org/1999/xlink" class="svglite" width="48.00pt" height="12.00pt" viewbox="0 0 48.00 12.00"><defs><style type="text/css">
#> .svglite line, .svglite polyline, .svglite polygon, .svglite path, .svglite rect, .svglite circle {
#> fill: none;
#> stroke: #000000;
#> stroke-linecap: round;
#> stroke-linejoin: round;
#> stroke-miterlimit: 10.00;
#> }
#> .svglite text {
#> white-space: pre;
#> }
#> </style></defs><rect width="100%" height="100%" style="stroke: none; fill: none;"></rect><defs><clippath id="cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw"><rect x="0.00" y="0.00" width="48.00" height="12.00"></rect></clippath></defs><g clip-path="url(#cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw)"><polyline points="3.42,6.00 11.40,6.00 " style="stroke-width: 7.50; stroke: #D3D3D3;"></polyline><text x="24.00" y="66.72" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="164.92px" lengthadjust="spacingAndGlyphs">c(0, 0.0300225008036001)</text><text transform="translate(-46.08,6.00) rotate(-90)" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="38.87px" lengthadjust="spacingAndGlyphs">c(1, 1)</text></g></svg>
#> </td>
#> <td style="text-align:right;"> -0.8464852 </td>
#> <td style="text-align:right;"> 1.3575986 </td>
#> <td style="text-align:right;"> -0.5710801 </td>
#> <td style="text-align:right;"> <span style=" border-radius: 4px; padding-right: 4px; padding-left: 4px; background-color: #F08080 !important;">0.53295</span> </td>
#> </tr>
#> <tr>
#> <td style="text-align:left;"> Cabintype </td>
#> <td style="text-align:left;"> Missing </td>
#> <td style="text-align:left;"> <svg xmlns="http://www.w3.org/2000/svg" xmlns:xlink="http://www.w3.org/1999/xlink" class="svglite" width="48.00pt" height="12.00pt" viewbox="0 0 48.00 12.00"><defs><style type="text/css">
#> .svglite line, .svglite polyline, .svglite polygon, .svglite path, .svglite rect, .svglite circle {
#> fill: none;
#> stroke: #000000;
#> stroke-linecap: round;
#> stroke-linejoin: round;
#> stroke-miterlimit: 10.00;
#> }
#> .svglite text {
#> white-space: pre;
#> }
#> </style></defs><rect width="100%" height="100%" style="stroke: none; fill: none;"></rect><defs><clippath id="cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw"><rect x="0.00" y="0.00" width="48.00" height="12.00"></rect></clippath></defs><g clip-path="url(#cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw)"><polyline points="3.42,6.00 11.40,6.00 " style="stroke-width: 7.50; stroke: #D3D3D3;"></polyline><text x="24.00" y="66.72" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="164.92px" lengthadjust="spacingAndGlyphs">c(0, 0.0300225008036001)</text><text transform="translate(-46.08,6.00) rotate(-90)" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="38.87px" lengthadjust="spacingAndGlyphs">c(1, 1)</text></g></svg>
#> </td>
#> <td style="text-align:right;"> -0.9716536 </td>
#> <td style="text-align:right;"> 0.6953293 </td>
#> <td style="text-align:right;"> -0.6215433 </td>
#> <td style="text-align:right;"> <span style=" border-radius: 4px; padding-right: 4px; padding-left: 4px; background-color: #F08080 !important;">0.16229</span> </td>
#> </tr>
#> <tr>
#> <td style="text-align:left;"> Cabintype </td>
#> <td style="text-align:left;"> T </td>
#> <td style="text-align:left;"> <svg xmlns="http://www.w3.org/2000/svg" xmlns:xlink="http://www.w3.org/1999/xlink" class="svglite" width="48.00pt" height="12.00pt" viewbox="0 0 48.00 12.00"><defs><style type="text/css">
#> .svglite line, .svglite polyline, .svglite polygon, .svglite path, .svglite rect, .svglite circle {
#> fill: none;
#> stroke: #000000;
#> stroke-linecap: round;
#> stroke-linejoin: round;
#> stroke-miterlimit: 10.00;
#> }
#> .svglite text {
#> white-space: pre;
#> }
#> </style></defs><rect width="100%" height="100%" style="stroke: none; fill: none;"></rect><defs><clippath id="cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw"><rect x="0.00" y="0.00" width="48.00" height="12.00"></rect></clippath></defs><g clip-path="url(#cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw)"><polyline points="3.42,6.00 11.40,6.00 " style="stroke-width: 7.50; stroke: #D3D3D3;"></polyline><text x="24.00" y="66.72" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="164.92px" lengthadjust="spacingAndGlyphs">c(0, 0.0300225008036001)</text><text transform="translate(-46.08,6.00) rotate(-90)" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="38.87px" lengthadjust="spacingAndGlyphs">c(1, 1)</text></g></svg>
#> </td>
#> <td style="text-align:right;"> -14.0675600 </td>
#> <td style="text-align:right;"> 882.7435793 </td>
#> <td style="text-align:right;"> -0.9999992 </td>
#> <td style="text-align:right;"> <span style=" border-radius: 4px; padding-right: 4px; padding-left: 4px; background-color: #F08080 !important;">0.98729</span> </td>
#> </tr>
#> <tr>
#> <td style="text-align:left;"> Sex:Fare </td>
#> <td style="text-align:left;"> female:Fare_0_250 </td>
#> <td style="text-align:left;"> <svg xmlns="http://www.w3.org/2000/svg" xmlns:xlink="http://www.w3.org/1999/xlink" class="svglite" width="48.00pt" height="12.00pt" viewbox="0 0 48.00 12.00"><defs><style type="text/css">
#> .svglite line, .svglite polyline, .svglite polygon, .svglite path, .svglite rect, .svglite circle {
#> fill: none;
#> stroke: #000000;
#> stroke-linecap: round;
#> stroke-linejoin: round;
#> stroke-miterlimit: 10.00;
#> }
#> .svglite text {
#> white-space: pre;
#> }
#> </style></defs><rect width="100%" height="100%" style="stroke: none; fill: none;"></rect><defs><clippath id="cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw"><rect x="0.00" y="0.00" width="48.00" height="12.00"></rect></clippath></defs><g clip-path="url(#cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw)"><polyline points="3.42,6.00 44.58,6.00 " style="stroke-width: 7.50; stroke: #D3D3D3;"></polyline><text x="24.00" y="66.72" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="157.28px" lengthadjust="spacingAndGlyphs">c(0, 0.154979642130076)</text><text transform="translate(-46.08,6.00) rotate(-90)" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="38.87px" lengthadjust="spacingAndGlyphs">c(1, 1)</text></g></svg>
#> </td>
#> <td style="text-align:right;"> 0.0744651 </td>
#> <td style="text-align:right;"> 0.0117103 </td>
#> <td style="text-align:right;"> 0.0773077 </td>
#> <td style="text-align:right;"> <span style=" border-radius: 4px; padding-right: 4px; padding-left: 4px; background-color: #FFFFFF !important;">0</span> </td>
#> </tr>
#> <tr>
#> <td style="text-align:left;"> Sex:Fare </td>
#> <td style="text-align:left;"> male:Fare_0_250 </td>
#> <td style="text-align:left;"> <svg xmlns="http://www.w3.org/2000/svg" xmlns:xlink="http://www.w3.org/1999/xlink" class="svglite" width="48.00pt" height="12.00pt" viewbox="0 0 48.00 12.00"><defs><style type="text/css">
#> .svglite line, .svglite polyline, .svglite polygon, .svglite path, .svglite rect, .svglite circle {
#> fill: none;
#> stroke: #000000;
#> stroke-linecap: round;
#> stroke-linejoin: round;
#> stroke-miterlimit: 10.00;
#> }
#> .svglite text {
#> white-space: pre;
#> }
#> </style></defs><rect width="100%" height="100%" style="stroke: none; fill: none;"></rect><defs><clippath id="cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw"><rect x="0.00" y="0.00" width="48.00" height="12.00"></rect></clippath></defs><g clip-path="url(#cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw)"><polyline points="3.42,6.00 44.58,6.00 " style="stroke-width: 7.50; stroke: #D3D3D3;"></polyline><text x="24.00" y="66.72" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="157.28px" lengthadjust="spacingAndGlyphs">c(0, 0.154979642130076)</text><text transform="translate(-46.08,6.00) rotate(-90)" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="38.87px" lengthadjust="spacingAndGlyphs">c(1, 1)</text></g></svg>
#> </td>
#> <td style="text-align:right;"> -0.0065196 </td>
#> <td style="text-align:right;"> 0.0049329 </td>
#> <td style="text-align:right;"> -0.0064984 </td>
#> <td style="text-align:right;"> <span style=" border-radius: 4px; padding-right: 4px; padding-left: 4px; background-color: #F08080 !important;">0.18628</span> </td>
#> </tr>
#> <tr>
#> <td style="text-align:left;"> Sex:Fare </td>
#> <td style="text-align:left;"> female:Fare_250_600 </td>
#> <td style="text-align:left;"> <svg xmlns="http://www.w3.org/2000/svg" xmlns:xlink="http://www.w3.org/1999/xlink" class="svglite" width="48.00pt" height="12.00pt" viewbox="0 0 48.00 12.00"><defs><style type="text/css">
#> .svglite line, .svglite polyline, .svglite polygon, .svglite path, .svglite rect, .svglite circle {
#> fill: none;
#> stroke: #000000;
#> stroke-linecap: round;
#> stroke-linejoin: round;
#> stroke-miterlimit: 10.00;
#> }
#> .svglite text {
#> white-space: pre;
#> }
#> </style></defs><rect width="100%" height="100%" style="stroke: none; fill: none;"></rect><defs><clippath id="cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw"><rect x="0.00" y="0.00" width="48.00" height="12.00"></rect></clippath></defs><g clip-path="url(#cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw)"><polyline points="3.42,6.00 43.37,6.00 " style="stroke-width: 7.50; stroke: #D3D3D3;"></polyline><text x="24.00" y="66.72" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="157.28px" lengthadjust="spacingAndGlyphs">c(0, 0.150439301403622)</text><text transform="translate(-46.08,6.00) rotate(-90)" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="38.87px" lengthadjust="spacingAndGlyphs">c(1, 1)</text></g></svg>
#> </td>
#> <td style="text-align:right;"> -0.0150270 </td>
#> <td style="text-align:right;"> 2.7914016 </td>
#> <td style="text-align:right;"> -0.0149147 </td>
#> <td style="text-align:right;"> <span style=" border-radius: 4px; padding-right: 4px; padding-left: 4px; background-color: #F08080 !important;">0.9957</span> </td>
#> </tr>
#> <tr>
#> <td style="text-align:left;"> Sex:Fare </td>
#> <td style="text-align:left;"> male:Fare_250_600 </td>
#> <td style="text-align:left;"> <svg xmlns="http://www.w3.org/2000/svg" xmlns:xlink="http://www.w3.org/1999/xlink" class="svglite" width="48.00pt" height="12.00pt" viewbox="0 0 48.00 12.00"><defs><style type="text/css">
#> .svglite line, .svglite polyline, .svglite polygon, .svglite path, .svglite rect, .svglite circle {
#> fill: none;
#> stroke: #000000;
#> stroke-linecap: round;
#> stroke-linejoin: round;
#> stroke-miterlimit: 10.00;
#> }
#> .svglite text {
#> white-space: pre;
#> }
#> </style></defs><rect width="100%" height="100%" style="stroke: none; fill: none;"></rect><defs><clippath id="cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw"><rect x="0.00" y="0.00" width="48.00" height="12.00"></rect></clippath></defs><g clip-path="url(#cpMC4wMHw0OC4wMHwwLjAwfDEyLjAw)"><polyline points="3.42,6.00 43.37,6.00 " style="stroke-width: 7.50; stroke: #D3D3D3;"></polyline><text x="24.00" y="66.72" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="157.28px" lengthadjust="spacingAndGlyphs">c(0, 0.150439301403622)</text><text transform="translate(-46.08,6.00) rotate(-90)" text-anchor="middle" style='font-size: 12.00px; font-family: "Liberation Sans";' textlength="38.87px" lengthadjust="spacingAndGlyphs">c(1, 1)</text></g></svg>
#> </td>
#> <td style="text-align:right;"> 0.0289567 </td>
#> <td style="text-align:right;"> 0.0622836 </td>
#> <td style="text-align:right;"> 0.0293800 </td>
#> <td style="text-align:right;"> <span style=" border-radius: 4px; padding-right: 4px; padding-left: 4px; background-color: #F08080 !important;">0.64199</span> </td>
#> </tr>
#> </tbody>
#> <tfoot><tr><td style="padding: 0; " colspan="100%">
#> <span style="font-style: italic;text-decoration: underline;">Goodness-of-Fit:</span> <sup></sup> AIC: 870.9 , Devience : 826.9 , Null Devience: 1182.8</td></tr></tfoot>
#> </table>