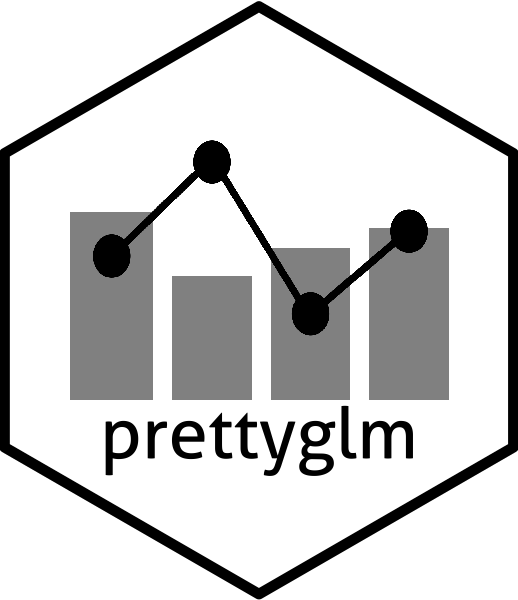
one_way_ave
one_way_ave.Rd
Creates a pretty html plot of one way actual vs expected by specified predictor.
Usage
one_way_ave(
feature_to_plot,
model_object,
target_variable,
data_set,
plot_type = "predictions",
plot_factor_as_numeric = FALSE,
ordering = NULL,
width = 800,
height = 500,
number_of_buckets = 30,
first_colour = "black",
second_colour = "#cc4678",
facetby = NULL,
prediction_type = "response",
predict_function = NULL,
upper_percentile_to_cut = 0.01,
lower_percentile_to_cut = 0
)
Arguments
- feature_to_plot
A string of the variable to plot.
- model_object
Model object to create coefficient table for. Must be of type: glm, lm
- target_variable
String of target variable name in dataset.
- data_set
Data set to calculate the actual vs expected for. If no input default is to try and extract training data from model object.
- plot_type
one of "Residual", "predictions" or "actuals" defaults to "predictions"
- plot_factor_as_numeric
Set to TRUE to return data.frame instead of creating kable.
- ordering
Option to change the ordering of categories on the x axis, only for discrete categories. Default to the ordering of the factor. Other options are: 'alphabetical', 'Number of records', 'Average Value'
- width
Width of plot
- height
Height of plot
- number_of_buckets
Number of buckets for continuous variable plots
- first_colour
First colour to plot, usually the colour of actual.
- second_colour
Second colour to plot, usually the colour of predicted.
- facetby
Variable to facet the actual vs expect plots by.
- prediction_type
Prediction type to be pasted to predict.glm if predict_function is NULL. Defaults to "response".
- predict_function
A custom prediction function can be provided here.It must return a data.frame with an "Actual_Values" column, and a "Predicted_Values" column.
- upper_percentile_to_cut
For continuous variables this is what percentile to exclude from the upper end of the distribution. Defaults to 0.01, so the maximum percentile of the variable in the plot will be 0.99. Cutting off some of the distribution can help the views if outlier's are present in the data.
- lower_percentile_to_cut
For continuous variables this is what percentile to exclude from the lower end of the distribution. Defaults to 0.01, so the minimum percentile of the variable in the plot will be 0.01. Cutting off some of the distribution can help the views if outlier's are present in the data.
Examples
library(dplyr)
library(prettyglm)
data('titanic')
columns_to_factor <- c('Pclass',
'Sex',
'Cabin',
'Embarked',
'Cabintype',
'Survived')
meanage <- base::mean(titanic$Age, na.rm=TRUE)
titanic <- titanic %>%
dplyr::mutate_at(columns_to_factor, list(~factor(.))) %>%
dplyr::mutate(Age =base::ifelse(is.na(Age)==TRUE,meanage,Age)) %>%
dplyr::mutate(Age_0_25 = prettyglm::splineit(Age,0,25),
Age_25_50 = prettyglm::splineit(Age,25,50),
Age_50_120 = prettyglm::splineit(Age,50,120)) %>%
dplyr::mutate(Fare_0_250 = prettyglm::splineit(Fare,0,250),
Fare_250_600 = prettyglm::splineit(Fare,250,600))
survival_model <- stats::glm(Survived ~
Sex:Age +
Fare +
Embarked +
SibSp +
Parch +
Cabintype,
data = titanic,
family = binomial(link = 'logit'))
# Continuous Variable Example
one_way_ave(feature_to_plot = 'Age',
model_object = survival_model,
target_variable = 'Survived',
data_set = titanic,
number_of_buckets = 20,
upper_percentile_to_cut = 0.1,
lower_percentile_to_cut = 0.1)
# Discrete Variable Example
one_way_ave(feature_to_plot = 'Pclass',
model_object = survival_model,
target_variable = 'Survived',
data_set = titanic)
# Custom Predict Function and facet
a_custom_predict_function <- function(target, model_object, dataset){
dataset <- base::as.data.frame(dataset)
Actual_Values <- dplyr::pull(dplyr::select(dataset, tidyselect::all_of(c(target))))
if(class(Actual_Values) == 'factor'){
Actual_Values <- base::as.numeric(as.character(Actual_Values))
}
Predicted_Values <- base::as.numeric(stats::predict(model_object, dataset, type='response'))
to_return <- base::data.frame(Actual_Values = Actual_Values,
Predicted_Values = Predicted_Values)
to_return <- to_return %>%
dplyr::mutate(Predicted_Values = base::ifelse(Predicted_Values > 0.3,0.3,Predicted_Values))
return(to_return)
}
one_way_ave(feature_to_plot = 'Age',
model_object = survival_model,
target_variable = 'Survived',
data_set = titanic,
number_of_buckets = 20,
upper_percentile_to_cut = 0.1,
lower_percentile_to_cut = 0.1,
predict_function = a_custom_predict_function,
facetby = 'Pclass')